REACTJS - Aplicação de Página �nica - #7 Informações e Mapa
27/10/2018Olá Pessoal. Faremos na vÃdeo-aula de hoje o componente Information e o componente Maps.
Informações e Mapas com ReactJS
O componente information ficará ao lado do slideshow e receberá os dados principais do imóvel:
import React from 'react';
export default class Information extends React.Component {
render(){
return(
<div>
<div className="information">
<ul>
<li>2 quartos</li>
<li>1 vaga de garagem</li>
<li>1 banheiro</li>
<li>Cozinha americana</li>
<li>Ampla sala</li>
<li>(...)</li>
</ul>
</div>
<div className="informationText">
Apartamento com dois quartos com piso em cerâmica, sala ampla, cozinha americana com piso e revestimento em cerâmica e bancada de granito, banho social com piso e revestido em cerâmica e área de serviço compartilhada bem arejada. Uma vaga de garagem demarcada e descoberta Portaria 24h. <br/>
Agende hoje mesmo uma visita e venha conhecer este imóvel. Preços poderão sofrer alterações sem aviso prévio, consulte condições com nosso departamento de locação.
</div>
</div>
);
}
}
O componente Maps fará um embed do Google Maps:
import React from 'react';
export default class Maps extends React.Component {
render(){
return(
<div className="mapsImovel">
<iframe src="https://www.google.com/maps/embed?pb=!1m18!1m12!1m3!1d3749.517439289106!2d-44.03480858508463!3d-19.986786386571918!2m3!1f0!2f0!3f0!3m2!1i1024!2i768!4f13.1!3m3!1m2!1s0xa6be65a53292b9%3A0xf61da899a0c0b9d9!2sR.+Me.+Paulina%2C+210+-+Diamante%2C+Belo+Horizonte+-+MG%2C+30660-630!5e0!3m2!1spt-BR!2sbr!4v1539554138845" allowFullScreen></iframe>
</div>
);
}
}
Estilizando os componentes e refatorando o código da última aula teremos o seguinte CSS:
/*General*/
*{margin: 0; padding: 0;-webkit-box-sizing: border-box;-moz-box-sizing: border-box;box-sizing: border-box; font-size: 18px; font-family: "Helvetica Neue", sans-serif; color:#333;}
a{text-decoration: none; font-weight: bold;}
.nav{background: #38777E;}
.nav a{text-align: center; padding: 10px 0; color:#fff;}
.nav a:hover{background: #51A8B1;}
.address{text-align: center;}
.address img{vertical-align: middle;}
.image-gallery, .information{float:left;}
.information li{list-style: none;line-height: 35px;padding-left: 20px;}
.mapsImovel{float:left; width: 100%; margin: 35px 0;}
.mapsImovel iframe{width: 100%; height: 400px; border:none;}
.informationText{float:left; width: 90%; margin: 30px 5%; line-height: 35px;}
/*Small Smartphones*/
@media screen and (max-width: 480px) {
.header{display:flex; background: #efefef; flex-direction: column; margin-bottom: 15px;}
.nav{display:none; flex-direction: column; order:2;}
.nav a{width: 100%; }
.buttonMob{order:1; text-align: right; padding: 7px 5% 7px 0;}
.logo{position: absolute; top: 0; left: 5%;}
.address{order:3; padding: 10px 5%; line-height: 30px; color:#fff; background: #51A8B1;}
.address strong{font-size: 27px;}
.image-gallery{width: 90%; margin: 0 5%;}
.information{width: 90%; margin: 20px 5%;}
.information li{background: url("/img/icon-imovel.png") no-repeat left center;}
}
/*Medium Smartphones*/
@media screen and (min-width: 481px) and (max-width: 768px) {
.header{display:flex; background: #efefef; flex-direction: column; margin-bottom: 15px}
.nav{display:none; flex-direction: column;}
.nav a{width: 100%;}
.nav{display:none; flex-direction: column; order:2;}
.nav a{width: 100%; }
.buttonMob{order:1; text-align: right; padding: 5px 5% 5px 0;}
.logo{position: absolute; top: 0; left: 5%;}
.address{order:3; padding: 10px 5%; line-height: 30px; color:#fff; text-align: center; background: #51A8B1;}
.address strong{font-size: 27px;}
.image-gallery{width: 80%; margin: 0 10%;}
.information{width: 80%; margin: 20px 10%;}
.information li{background: url("/img/icon-imovel.png") no-repeat left center;}
}
/*Large Smartphones and small notebooks*/
@media screen and (min-width: 769px) and (max-width: 1199px) {
.header{float:left; width: 100%; background: #efefef; box-shadow: 0 2px 10px #999999; margin-bottom: 25px;}
.nav{display:flex;}
.nav a{width: 25%;}
.buttonMob{display:none;}
.logo{float:left; width: 30%; padding: 15px 0 15px 10%;}
.address{float:left; width: 70%; padding: 20px 5% 10px 20%; line-height: 35px; text-align: center;}
.address strong{color: #51A8B1; font-size: 27px;}
.image-gallery{width: 52%; margin: 0 4%;}
.information{width: 40%;}
.information li{background: url("/img/icon-imovel.png") no-repeat left center;}
}
/*XLarges screens*/
@media screen and (min-width: 1200px) {
.header{float:left; width: 100%; background: #efefef; box-shadow: 0 2px 10px #999999; margin-bottom: 25px;}
.nav{display:flex;}
.nav a{width: 25%;}
.buttonMob{display:none;}
.logo{float:left; width: 30%; padding: 15px 0 15px 10%;}
.address{float:left; width: 70%; padding: 20px 5% 10px 20%; line-height: 35px; text-align: center;}
.address strong{color: #51A8B1; font-size: 27px;}
.image-gallery{width: 36%; margin: 0 7%;}
.information{width: 50%;}
.information li{background: url("/img/icon-imovel.png") no-repeat left center;}
.mapsImovel iframe{height: 400px;}
}

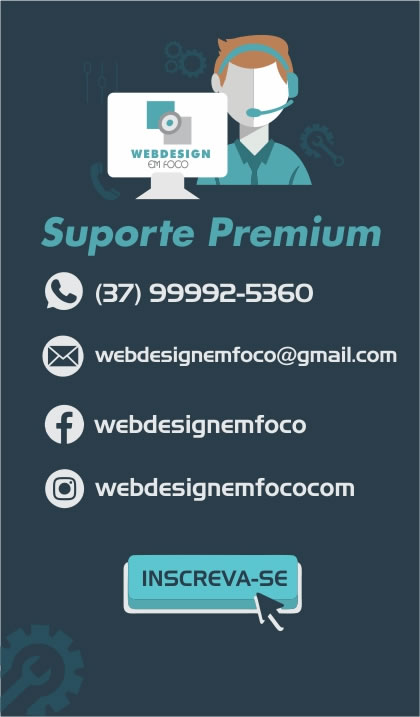
Posts Relacionados
REACTJS - Aplicação de Página �nica - #6 Slideshow
No vÃdeo-tutorial de hoje vamos criar o slideshow do nosso projeto. Para tanto vamos editar o componente Slide.js.
REACTJS - Aplicação de Página �nica - #8 Footer
Hoje faremos a construção e estilização do componente footer do nosso website.