Calendário / Agenda com PHP & JS - #11 Editando Eventos
10/12/2021Nesta aula aprenderemos como realizar a edição de eventos diretamente no banco de dados mysql, fazendo um update na tabela de eventos através do PHP e Ajax.
Update de Eventos no Banco MySQL
Para realizarmos a modificação de um evento, usaremos javascript e PHP.
lib/js/javascript.js
No Javascript vamos trabalhar no método dateClick e eventClick.
(function(win,doc){
'use strict';
//Exibir o calendário
function getCalendar(perfil, div)
{
let calendarEl=doc.querySelector(div);
let calendar = new FullCalendar.Calendar(calendarEl, {
initialView: 'dayGridMonth',
headerToolbar:{
start: 'prev,next,today',
center: 'title',
end: 'dayGridMonth, timeGridWeek, timeGridDay'
},
buttonText:{
today: 'hoje',
month: 'mês',
week: 'semana',
day: 'dia'
},
locale:'pt-br',
dateClick: function(info) {
if(perfil == 'manager'){
calendar.changeView('timeGrid', info.dateStr);
}else{
if(info.view.type == 'dayGridMonth'){
calendar.changeView('timeGrid', info.dateStr);
}else{
win.location.href='/views/user/add.php?date='+info.dateStr;
}
}
},
events: '/controllers/ControllerEvents.php',
eventClick: function(info) {
if(perfil == 'manager'){
win.location.href=`/views/manager/editar.php?id=${info.event.id}`;
}
}
});
calendar.render();
}
if(doc.querySelector('.calendarUser')){
getCalendar('user','.calendarUser');
}else if(doc.querySelector('.calendarManager')){
getCalendar('manager','.calendarManager');
}
})(window,document);
views/manager/editar.php
Dentro da pasta manager vamos criar o arquivo responsável por recepcionar o formulário de edição:
<?php include("../../config/config.php"); ?>
<?php include(DIRREQ."lib/html/header.php"); ?>
<?php
$objEvents=new \Classes\ClassEvents();
$events=$objEvents->getEventsById($_GET['id']);
$date=new \DateTime($events['start']);
?>
<form name="formEdit" id="formEdit" method="post" action="<?php echo DIRPAGE.'controllers/ControllerEdit.php'; ?>">
<input type="hidden" name="id" id="id" value="<?php echo $_GET['id']; ?>"><br>
Data: <input type="date" name="date" id="date" value="<?php echo $date->format("Y-m-d"); ?>"><br>
Hora: <input type="time" name="time" id="time" value="<?php echo $date->format("H:i"); ?>"><br>
Paciente: <input type="text" name="title" id="title" value="<?php echo $events['title']; ?>"><br>
Queixa: <input type="text" name="description" id="description" value="<?php echo $events['description']; ?>"><br>
<input type="submit" value="Confirmar Consulta">
</form>
<?php include(DIRREQ."lib/html/footer.php"); ?>
class/ClassEvents.php
Na classe de evento vamos criar o método que vai retornar o evento pelo id e outro que irá fazer o update no banco:
#Buscar eventos pelo id
public function getEventsById($id)
{
$b=$this->conectDB()->prepare("select * from events where id=?");
$b->bindParam(1, $id, \PDO::PARAM_INT);
$b->execute();
return $f=$b->fetch(\PDO::FETCH_ASSOC);
}
#Update no banco de dados
public function updateEvent($id,$title,$description,$start)
{
$b=$this->conectDB()->prepare("update events set title=?, description=?, start=? where id=?");
$b->bindParam(1, $title, \PDO::PARAM_STR);
$b->bindParam(2, $description, \PDO::PARAM_STR);
$b->bindParam(3, $start, \PDO::PARAM_STR);
$b->bindParam(4, $id, \PDO::PARAM_INT);
$b->execute();
}
controllers/ControllerEdit.php
Vamos criar também o controller para edição dos eventos:
<?php
include ("../config/config.php");
$objEvents=new \Classes\ClassEvents();
$date=filter_input(INPUT_POST,'date',FILTER_DEFAULT);
$time=filter_input(INPUT_POST,'time',FILTER_DEFAULT);
$id=filter_input(INPUT_POST,'id',FILTER_DEFAULT);
$title=filter_input(INPUT_POST,'title',FILTER_DEFAULT);
$description=filter_input(INPUT_POST,'description',FILTER_DEFAULT);
$start=new \DateTime($date.' '.$time, new \DateTimeZone('America/Sao_Paulo'));
$objEvents->updateEvent(
$id,
$title,
$description,
$start->format("Y-m-d H:i:s")
);
Sucesso nos códigos e na vida!
Precisa de aulas particulares? webdesignemfoco@gmail.com

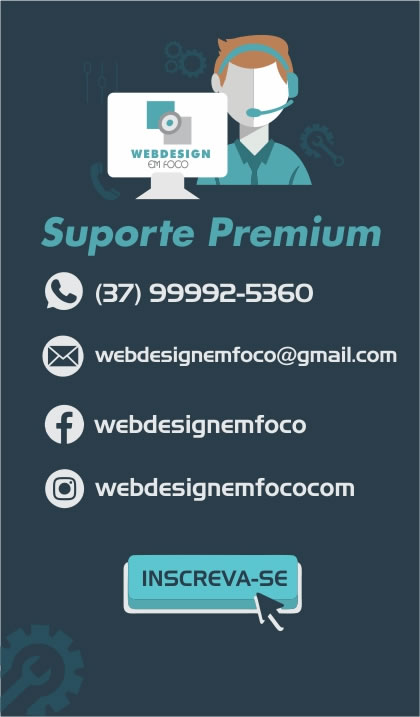
Posts Relacionados
Calendário / Agenda com PHP & JS - #10 Cadastrando Eventos no Banco
No tutorial de hoje aprenderemos como inserir novos eventos no banco de dados através de um formulário html.
Calendário / Agenda com PHP & JS - #12 Deletando Eventos
Neste tutorial vamos trabalhar com a parte de deletar eventos do calendário, eliminando os registros do banco de dados.