Vanilla Javascript - #44 Bubbling e Capturing
13/10/2019Nessa videoaula iremos aprender dois conceitos importantes e básicos para todos os programadores Javascript, conheceremos o bubbling e o capturing.
Bubbling e Capturing no JS
Como visto na imagem acima, o bubbling é o sistema padrão do JS e segundo ele, os eventos iniciam pelo elemento filho subindo como bolhas para o elemento pai. O capturing é justamente o contrário.
Repare que nesse exemplo, quando clicamos na div, o Javascript irá ler primeiro a div, subindo até o document do DOM.
Vamos ver esses dois conceitos na prática agora. Criaremos o arquivo index.php, javascript.js e style.css. Eles ficaram assim:
index.php
<!doctype html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport"
content="width=device-width, user-scalable=no, initial-scale=1.0, maximum-scale=1.0, minimum-scale=1.0">
<meta http-equiv="X-UA-Compatible" content="ie=edge">
<title>Document</title>
<link rel="stylesheet" href="style.css">
</head>
<body>
<div class="parent">
<div class="child">
<div class="grandchild">
<form id="form1" action="">
<input type="text" name="search" id="search">
</form>
</div>
</div>
</div>
<script src="javascript.js"></script>
</body>
</html>
style.css
.parent{
width: 200px;
height: 200px;
background: green;
}
.child{
width: 50px;
height: 50px;
background: red;
}
.grandchild{
width: 10px;
height: 10px;
background: blue;
}
javascript.js
(function (win,doc) {
'use strict';
let parent=doc.querySelector('.parent');
let child=doc.querySelector('.child');
let grandchild=doc.querySelector('.grandchild');
let form=doc.querySelector('#form1');
/*parent.addEventListener('click',function () {
console.log('parent');
},false);
child.addEventListener('click',function () {
console.log('child');
},false);
grandchild.addEventListener('click',function () {
console.log('grandchild');
},false);*/
/*parent.addEventListener('click',function (event) {
console.log('this',this);
console.log('event',event.target);
},false);*/
/*parent.addEventListener('click',function (event) {
console.log('parent');
},false);
child.addEventListener('click',function () {
console.log('child');
},false);
grandchild.addEventListener('click',function (event) {
event.stopPropagation();
console.log('grandchild');
},false);*/
form.addEventListener('focus',function(){
alert('oi');
},true);
})(window,document);
Por hoje é só! Sucesso nos códigos e na vida!

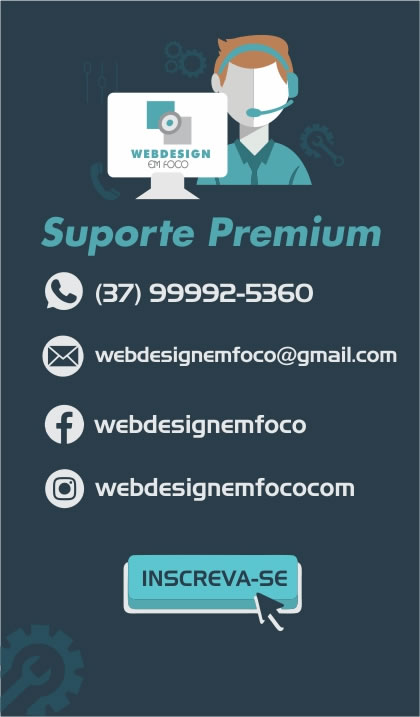
Posts Relacionados
Vanilla Javascript - #43 Função debounce
Na vídeo aula de hoje trataremos de uma função muito importante principalmente quando temos requisições sucessivas tais como as utilizadas no evento keyup.
Vanilla Javascript - #45 Call, Apply e Bind
Neste tutorial iremos tratar dos métodos manipuladores do this, dentre eles veremos as funções: call, apply e bind.